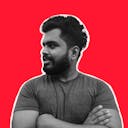
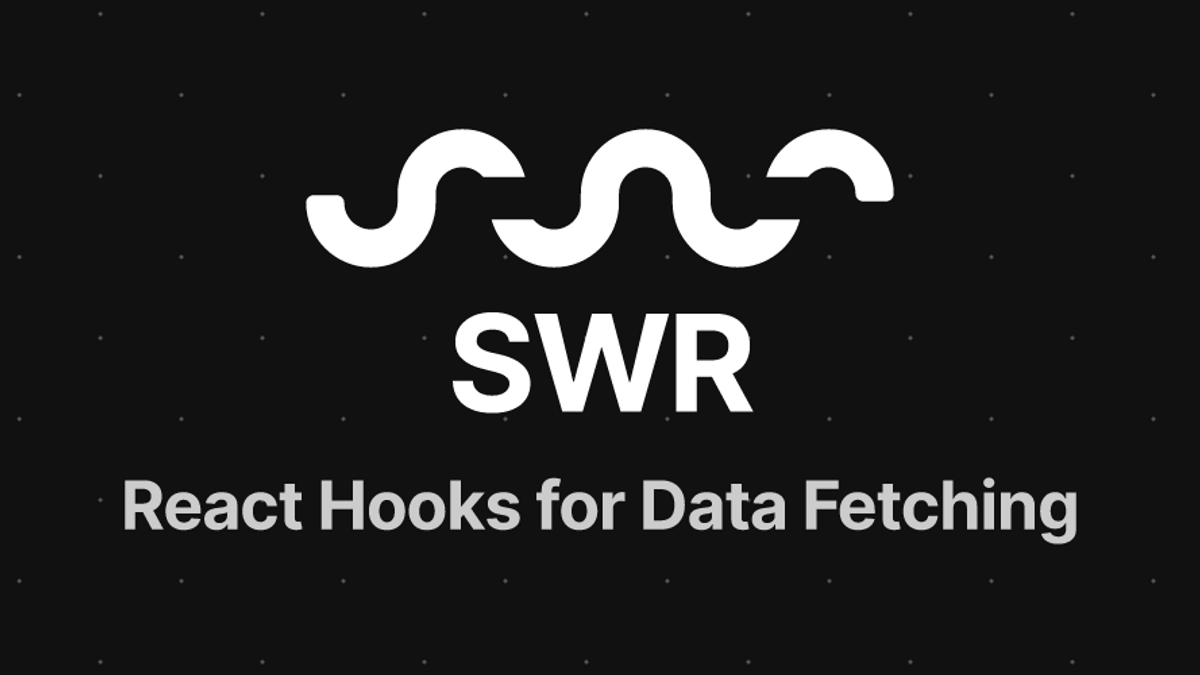
SWR, short for Stale While Revalidate, is a caching strategy designed to enhance the speed and responsiveness of web applications. It is beneficial in scenarios where maintaining up-to-date data is crucial while minimising the impact on the user experience.
How does SWR work?
The key idea behind SWR is to serve stale (outdated) data immediately to the user while concurrently fetching the latest data from the server in the background. SWR has two approches:
1. Stale
When a user requests the data, the cached response will be served immediately, even if it is slightly outdated. This is called the “stale” part of SWR. This stale data will ensure quick response time, improving the application's performance.
2. Revalidate
Simultaneously, a request will be sent to the server to fetch the latest data. This is called revalidation in SWR. After receiving the updated data, SWR updates the cache with the most current information. This helps data remain relatively fresh, even if the user is not interacting with the application.
Advantages of SWR
- Improved Performance - Users will experience faster load times as the stale data is served immediately.
- Reduced Server Load - By serving stale data while revalidating in the background, SWR minimizes the load on the server during peak usage times.
Disadvantages of SWR
- SWR has a few disadvantages, but the major one is stale data exposure, which means displaying slightly outdated information. This leads to an inaccurate user experience.
- Another disadvantage is the limited applicability of SWR; this may not be suitable for all types of applications. For example, for financial applications real-time data Is critical. So, it's not a great situation to use SWR to fetch data.
How to Use SWR?
1. Install SWR:
You can install the SWR library using a package manager like npm or yarn. For example:
npm install swr
# or
yarn add swr
2. Define a Fetcher Function:
Create a function that fetches your data. SWR will use this function to request and refresh data.
//fetcher function
const fetcher = (...args) => fetch(...args).then(res => res.json())
4. Use SWR Hook:
Use the useSWR
hook in your component, passing in the key and the fetcher function:
import useSWR from 'swr'
function Profile () {
const fetcher = (...args) => fetch(...args).then(res => res.json())
const { data, error, isLoading } = useSWR('/api/user/123', fetcher)
if (error) return <div>failed to load</div>
if (isLoading) return <div>loading...</div>
// render data
return <div>hello {data.name}!</div>
}
More : SWR Docs
Share: